Using an authentication server
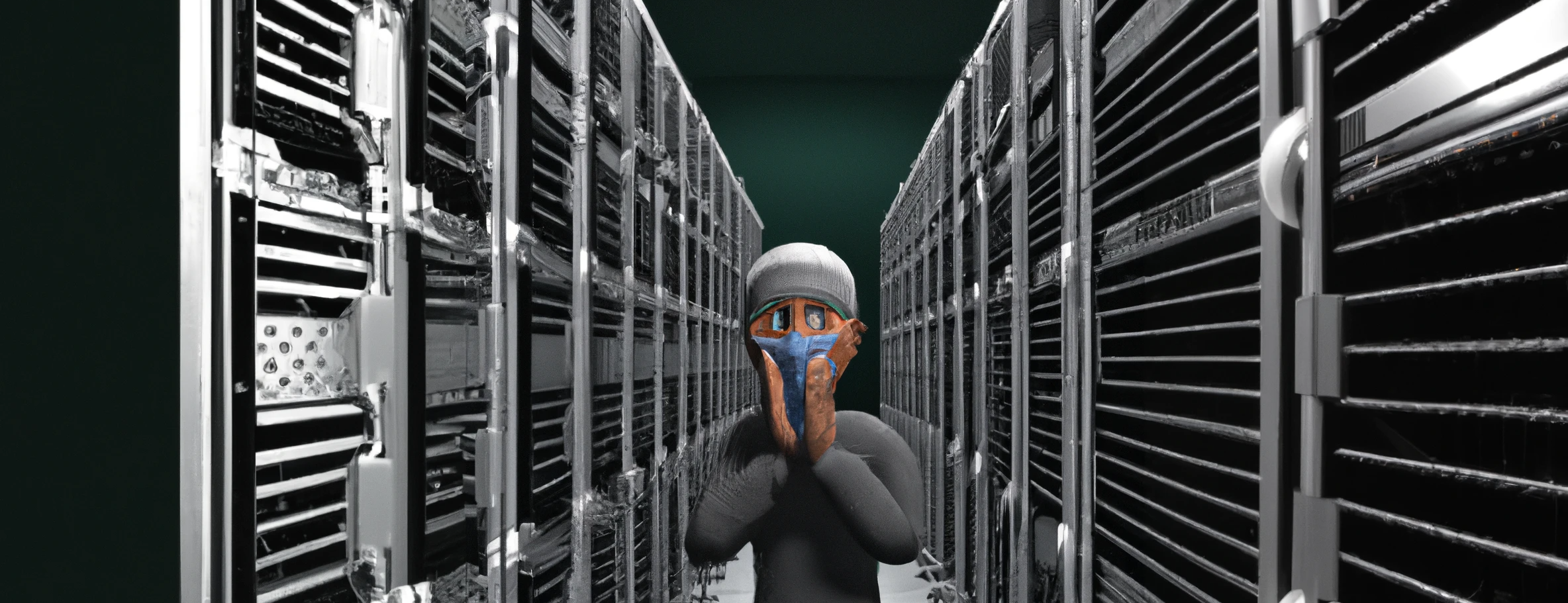
The easiest way to use OAuth2 with EmailEngine is to use the hosted authentication form feature. EmailEngine would take care of getting user permissions and renewing access tokens. Having EmailEngine managing everything is not always desirable. For example, you already use OAuth2 integration in other parts of your app and do not want to ask the user for permission twice.
If you do not want to use EmailEngine's OAuth2 flow but want to manage everything yourself, then there are some options. Perhaps the most obvious one would be to use the "authentication server." With the authentication server, you provide a web interface from which EmailEngine would ask for access tokens whenever it needs to authenticate an account. This process is completely transparent for users who never need to access the EmailEngine's UI.
The following uses Outlook as the example OAuth2 provider. Gmail follows a similar pattern.
- Ensure that your Azure app includes the following scopes:
IMAP.AccessAsUser.All
,SMTP.Send
- Make sure that when redirecting the user to Microsoft's sign-in page, you include the following
scope
values:https://outlook.office.com/IMAP.AccessAsUser.All
,https://outlook.office.com/SMTP.Send
- Create a web interface that takes the account id as the input and returns a currently valid access token as the response. This web interface would be the so-called "authentication server". See this example for the test implementation.
- Update EmailEngine's settings, set
authServer
value to the URL of your authentication server:
curl -XPOST "https://ee.example.com/v1/settings' \
-H 'Content-Type: application/json' \
-d '{
"authServer": "https://myservice.com/authentication"
}'
- When registering accounts via the API, do not set any authentication information, instead set
useAuthServer:true
curl -XPOST "https://ee.example.com/v1/account' \
-H 'Content-Type: application/json' \
-d '{
"account": "example",
"name": "My Email Account",
"email": "[email protected]",
"imap": {
"useAuthServer": true,
"host": "outlook.office365.com",
"port": 993,
"secure": true
},
"smtp": {
"useAuthServer": true,
"host": "smtp-mail.outlook.com",
"port": 587,
"secure": false
}
}'
- Whenever EmailEngine needs to authenticate that user, it makes an HTTP requests to your "authentication server" and provides the account id as the query argument. Whatever your server returns for the authentication info (which should include the access token) will then be used to authenticate that connection.
In general, the protocol for the authentication server is the following:
Request:
curl "https://myservice.com/authentication?account=example"
Response:
Content-type: application/json
{
"user": "[email protected]",
"accessToken": "tfhsgdfbsdjmfndsg......."
}
The provided accessToken
must be currently valid and not expired. How exactly do you ensure it is up to your app that manages these tokens.
This way, your users do not need to know anything about EmailEngine, as it would work completely in the background.
NB! make sure that the "authentication server" interface would not be publicly accessible. E.g., you use the firewall to allow requests only from specific IP addresses (the EmailEngine server), or you include a basic authentication username:password
in the authentication server's URL.