Sending replies and forwarding emails
While EmailEngine (a headless email client that allows accessing IMAP and SMTP accounts over REST API) can send regular emails where you provide all the details for the message yourself (recipients, subject line, and so on), two special modes help to achieve email-client-like behavior. These are "reply" and "forward" email actions. You can enable these modes by referencing an existing email on the account – the message you want to reply to or forward.
EmailEngine sends all emails using the SMTP server of the email account you are sending emails as. Sometimes users confuse EmailEngine as a general MTA server. This is not the case. EmailEngine never delivers any emails directly to MX servers.
You can do this by providing a reference
property for the message structure. Minimally you would then have to provide the ID of the message you are referencing and what to do with that message (either "reply" or "forward").
{
"reference": {
"message": "AAAADQAABl0",
"action": "reply"
},
...
}
Replies
When you reply to a referenced message, there are the following things to consider.
from
is not required. EmailEngine will fill it with the name and email address of the account that sends the email.to
is not required. EmailEngine will use the address from theReply-To
orFrom
headers of the referenced message.subject
is not required. EmailEngine will use the subject line of the referenced messages and if needed, adds a "Re: " prefix to it.- EmailEngine will add the
\Answered
flag to the referenced message in the IMAP server to get that arrow flag in email UIs.

- EmailEngine will add all the required
In-Reply-To
andReferences
headers, so you do not have to worry about these. Email clients use this information to bundle different emails into the same thread. - When using
reference.inline=true
option, EmailEngine will inline the contents of the referenced message as a cite. This mimics the behavior of regular email clients.
As so much is handled by EmailEngine, all you must provide is the message contents. Here is a minimally complete example for sending an email reply.
curl -XPOST "https://emailengine.example.com/v1/account/example/submit" \
-H "Content-type: application/json" \
-H "Authorization: Bearer 9e4dc192bdbc...838bd2ad" \
-d '{
"reference": {
"message": "AAAADQAABl0",
"inline": true,
"action": "reply"
},
"html": "Hello from myself!"
}'
Here's how this email looks in an email client. Note the inlined text "See on manustega kiri." This is the contents of the referenced email. EmailEngine fetched this from the IMAP server and embedded it in the sent email. Use inline:false
if you do not want to use such embedding.
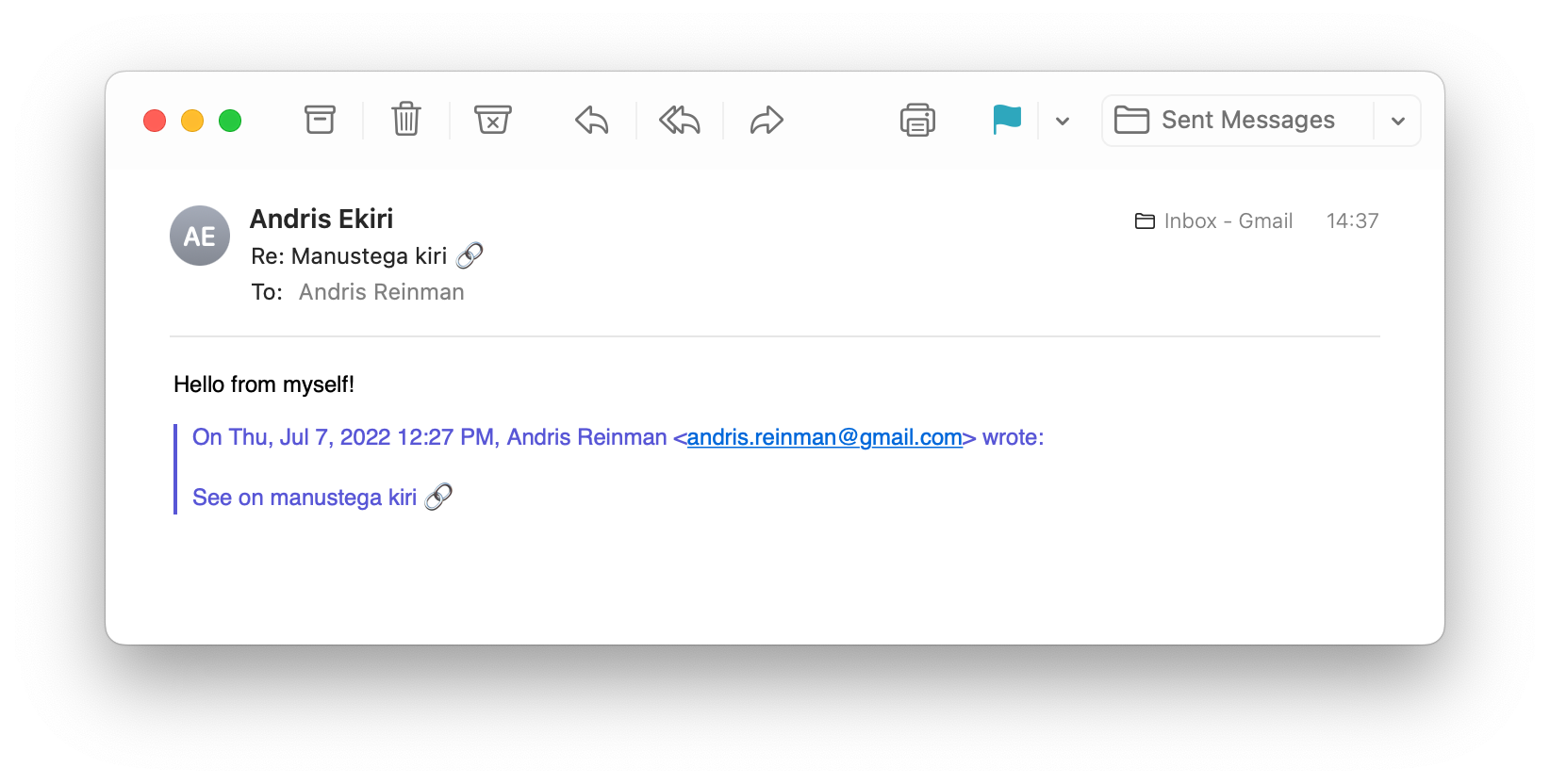
Forwarding
When forwarding a referenced message, there are the following things to consider.
from
is not required. EmailEngine will fill it with the name and email address of the account that sends the email.to
is required. Otherwise, EmailEngine would not know where to send that email.subject
is not required. EmailEngine will use the subject line of the referenced messages and if needed, adds an "Fwd: " prefix to it.- EmailEngine will add the
\Answered
flag to the referenced message in the IMAP server. - EmailEngine will add the required
References
header, so you do not have to worry about it. - When using
reference.inline=true
option, EmailEngine will inline the contents of the referenced message as a cite. This mimics the behavior of regular email clients. - When using
reference.forwardAttachments=true
option, EmailEngine will fetch the attachments from the email server and add these to the forwarded email.
Here is a minimally complete example for forwarding an email. It's a bit longer than replies as this time we have to provide the recipient info.
curl -XPOST "https://emailengine.example.com/v1/account/example/submit" \
-H "Content-type: application/json" \
-H "Authorization: Bearer 9e4dc192bdbc...838bd2ad" \
-d '{
"reference": {
"message": "AAAADQAABl0",
"inline": true,
"action": "forward",
"forwardAttachments": true
},
"to": {
"name":"Andris Reinman",
"address": "[email protected]"
},
"html": "Hello from myself!"
}'
As you can see, the resulting email looks slightly different than the reply, even though we were referencing the same email.
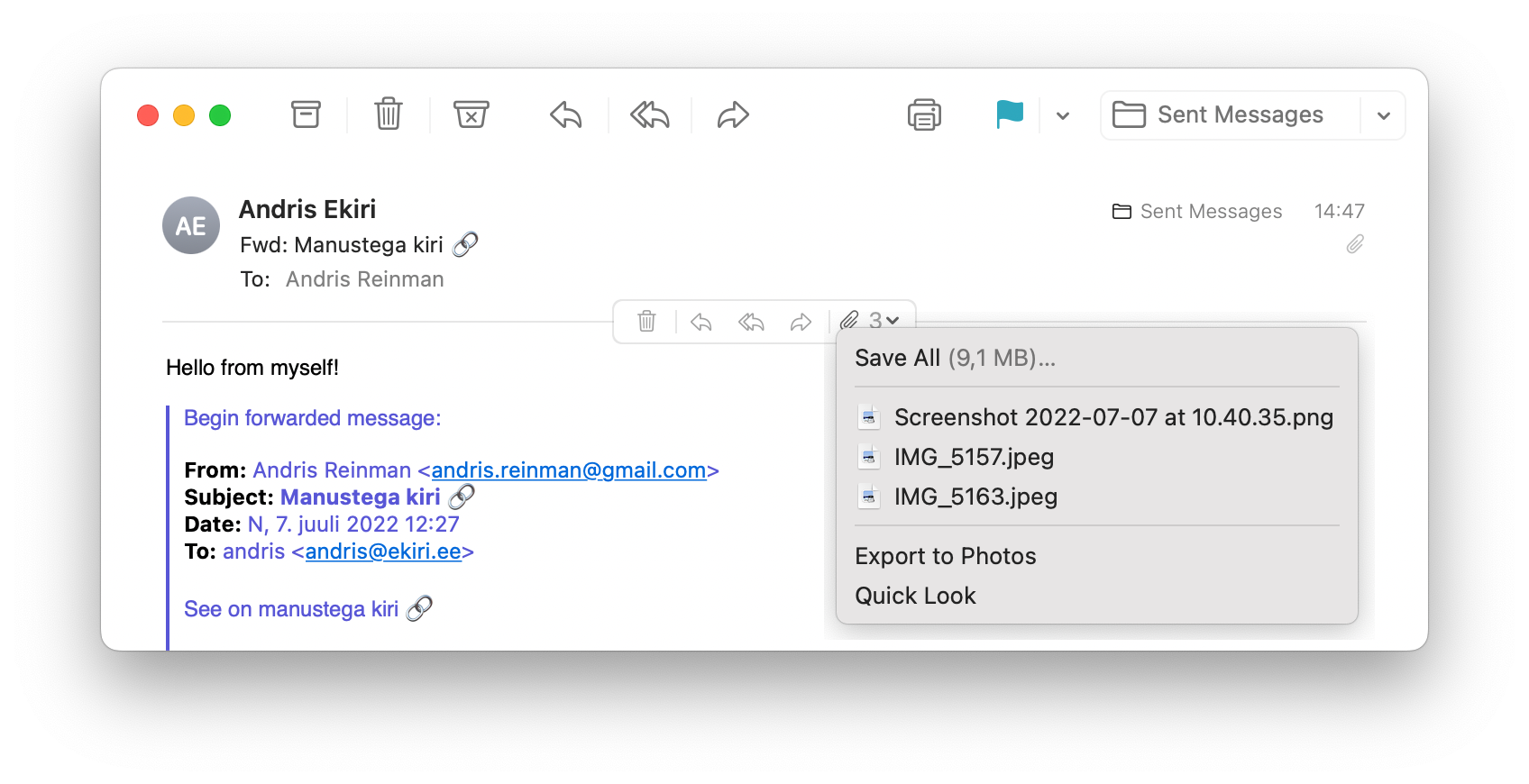
The inlined message includes a header section that was missing in the reply. Also, as we used forwardAttachments:true
then EmailEngine added all the attachments from the original message to the forwarded message.
You can use all these goodies starting with EmailEngine version 2.23.2. Happy sending!